Given two string arrays word1 and word2, return true if the two arrays represent the same string, and false otherwise. A string is represented by an array if the array elements concatenated in order forms the string.
Example 1:
Input: word1 = [“ab”, “c”], word2 = [“a”, “bc”]
Output: true
Explanation:
word1 represents string “ab” + “c” -> “abc”
word2 represents string “a” + “bc” -> “abc”
The strings are the same, so return true.
Flow Diagram: –
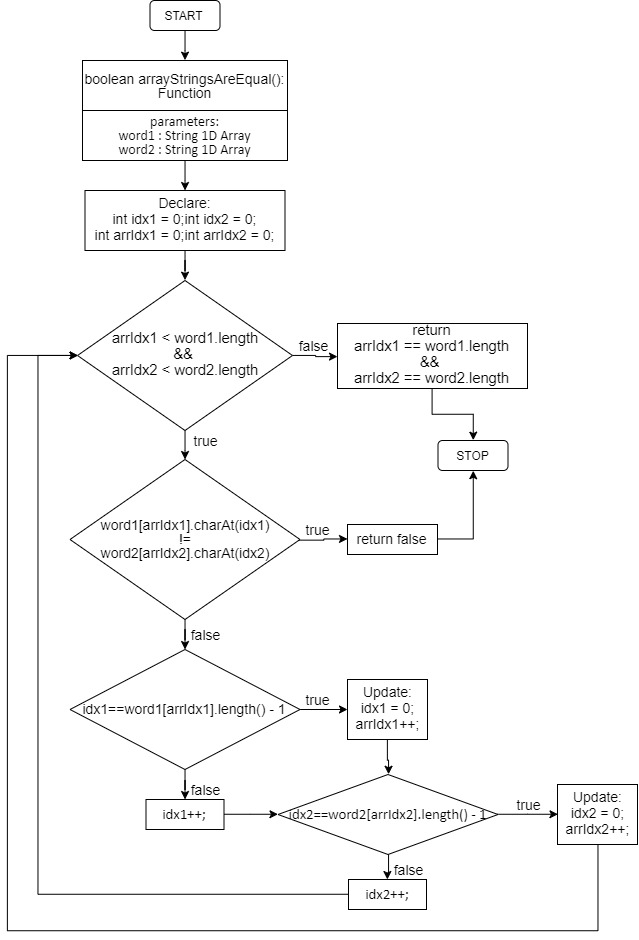
Solution: –
class Solution {
public boolean arrayStringsAreEqual(String[] word1, String[] word2) {
int idx1 = 0, idx2 = 0, arrIdx1 = 0, arrIdx2 = 0;
while (arrIdx1 < word1.length && arrIdx2 < word2.length) {
if (word1[arrIdx1].charAt(idx1) != word2[arrIdx2].charAt(idx2)) return false;
if (idx1 == word1[arrIdx1].length() - 1) {
idx1 = 0;
arrIdx1++;
} else idx1++;
if (idx2 == word2[arrIdx2].length() - 1) {
idx2 = 0;
arrIdx2++;
} else idx2++;
}
return arrIdx1 == word1.length && arrIdx2 == word2.length;
}
}