Given two n x n binary matrices mat and target, return true if it is possible to make mat equal to target by rotating mat in 90-degree increments, or false otherwise.
Example 1:
Input: mat = [[0,1],[1,0]], target = [[1,0],[0,1]]
Output: true
Explanation: We can rotate mat 90 degrees clockwise to make mat equal target.
Example 2:
Input: mat = [[0,1],[1,1]], target = [[1,0],[0,1]]
Output: false
Explanation: It is impossible to make mat equal to target by rotating mat.
Example 3:
Input: mat = [[0,0,0],[0,1,0],[1,1,1]], target = [[1,1,1],[0,1,0],[0,0,0]]
Output: true
Explanation: We can rotate mat 90 degrees clockwise two times to make mat equal target.
Constraints:
n == mat.length == target.length
n == mat[i].length == target[i].length
1 <= n <= 10
mat[i][j] and target[i][j] are either 0 or 1.
Flow Diagram: –
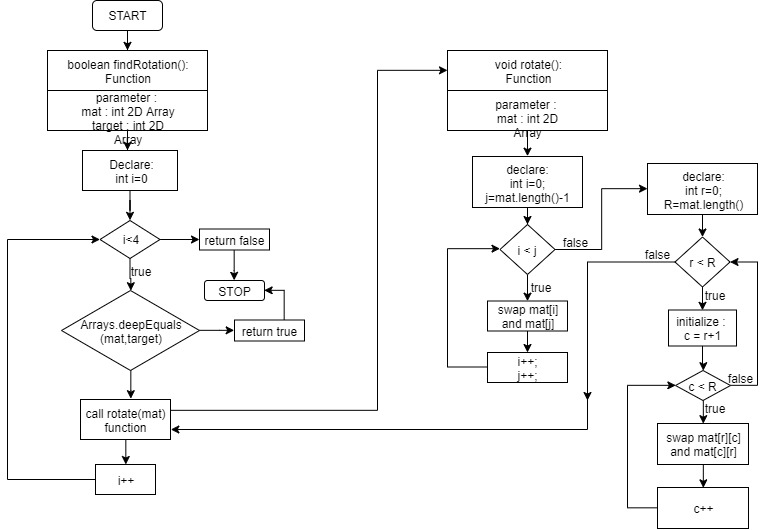
Solution: –
public boolean findRotation(int[][] mat, int[][] target) {
for (int i = 0; i < 4; ++i) { // rotate 0, 1, 2, 3 times.
if (Arrays.deepEquals(mat, target)) {
return true;
}
rotate(mat);
}
return false;
}
private void rotate(int[][] mat) {
for (int i = 0, j = mat.length - 1; i < j; ++i, --j) { // reverse rows order.
int[] tmp = mat[i];
mat[i] = mat[j];
mat[j] = tmp;
}
for (int r = 0, R = mat.length; r < R; ++r) { // transpose.
for (int c = r + 1; c < R; ++c) {
int tmp = mat[r][c];
mat[r][c] = mat[c][r];
mat[c][r] = tmp;
}
}
}