So for that at first we have to set the system property “webdriver.chrome.driver” and need to provide the path of the executable file of the chrome driver. The code block is mentioned below:-
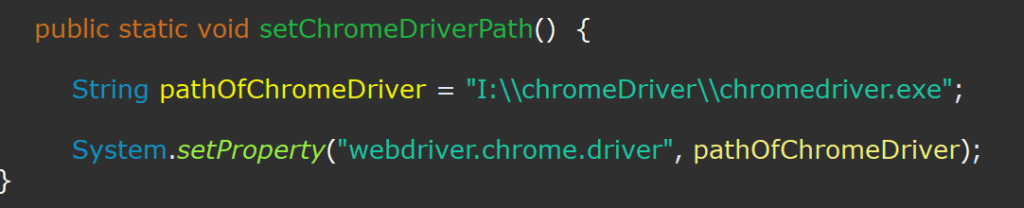
Now we can set the options of the chrome driver. This step is optional. Here we can set the execution mode of the chrome driver whether it should be headless or not and also the window sizes etc. Sample code is :-
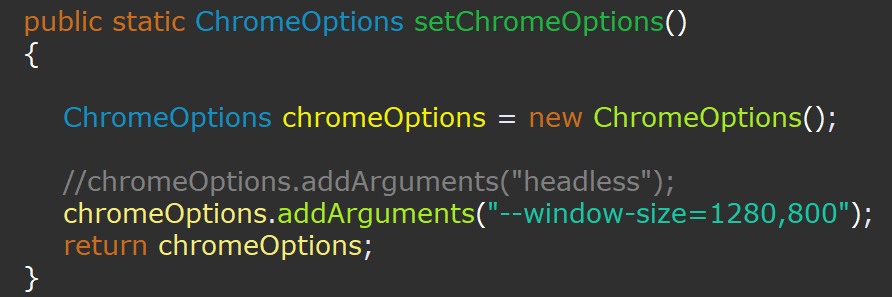
The source code for the “ChromeOptions” class can be found by clicking here. In the above mentioned code we have used the method “addArguments”. Which is the part of “ChromiumOptions” class. “ChromeOptions” class can access this method by extending the “ChromiumOptions” class. The source code of the “ChromiumOptions” class can be found here.
If we check the “ChromiumOptions” class, then we can find that it overloaded the add arguments method with two different signature. The code is copied from the source website only.
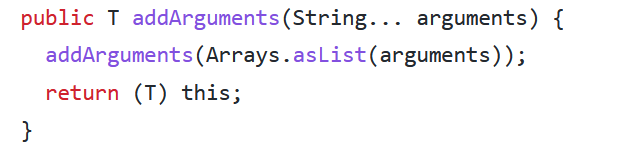
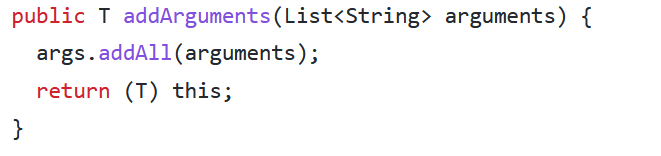
The first one takes the variable arguments and the second method takes the lists of Strings as arguments.
Now, at the time of initiation of the driver we can use the below mentioned code :-
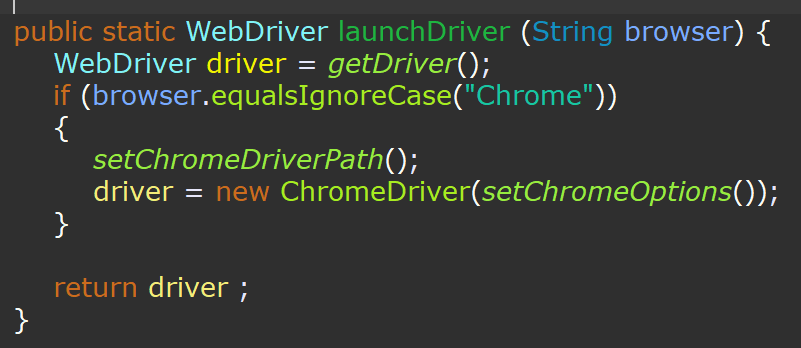
Here we are using different static methods. One is to set the property for the driver and another one is to set the “chromeOptions” for better code maintainability. Entire code block is attached below:-
package rest;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
public class SeleniumUtility {
static WebDriver driver = null;
public static WebDriver getDriver() {
return driver;
}
public static void setChromeDriverPath() {
String driverPath = "I:\\chromeDriver\\chromedriver.exe";
System.setProperty("webdriver.chrome.driver",driverPath);
}
public static ChromeOptions setChromeOptions()
{
ChromeOptions chromeOptions = new ChromeOptions();
//chromeOptions.addArguments("headless");
chromeOptions.addArguments("--window-size=1280,800");
return chromeOptions;
}
public static WebDriver launchDriver (String browser) {
WebDriver driver = getDriver();
if (browser.equalsIgnoreCase("Chrome"))
{
setChromeDriverPath();
driver = new ChromeDriver(setChromeOptions());
}
return driver ;
}
}
And for opening the desired website just extend this utility class as mentioned below:-
package rest;
import java.util.List;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class GoogleDynamicSearch extends SeleniumUtility {
public static void main(String[] args) throws InterruptedException {
//Set the google property
//System.setProperty("webdriver.chrome.driver", "I:\\chromeDriver\\chromedriver.exe");
//WebDriver driver = new ChromeDriver();
WebDriver driver = launchDriver("Chrome");
driver.get("https://www.google.com");
//Now type the selenium word in the search box
driver.findElement(By.name("q")).sendKeys("selenium");
Thread.sleep(1000);
////descendant::div[@class='wM6W7d']
WebElement listsDescendants = driver.findElement(By.xpath("//ul[@class='G43f7e']"));
List<WebElement> lists = listsDescendants.findElements(By.xpath("//li//span"));
// List<WebElement> lists = driver.findElements(By.xpath("//li[@class='sbct']//descendant"));
System.out.println(lists.size());
for(WebElement list:lists)
{
String text=list.getText();
if (text.equalsIgnoreCase("selenium interview questions"))
{
list.click();
break;
}
System.out.println(text);
}
//List <WebElement> listOfSuggestions = driver.findElement(By.xpath("//ul[@class='G43f7e']//li"));
//System.out.println("Prinit the sizes of suggestions >>>>>" + listOfSuggestions.size());
}
//set the chrome property
}