Maximum Subarray
Given an integer array nums
, find the contiguous subarray (containing at least one number) which has the largest sum and return its sum.
A subarray is a contiguous part of an array.
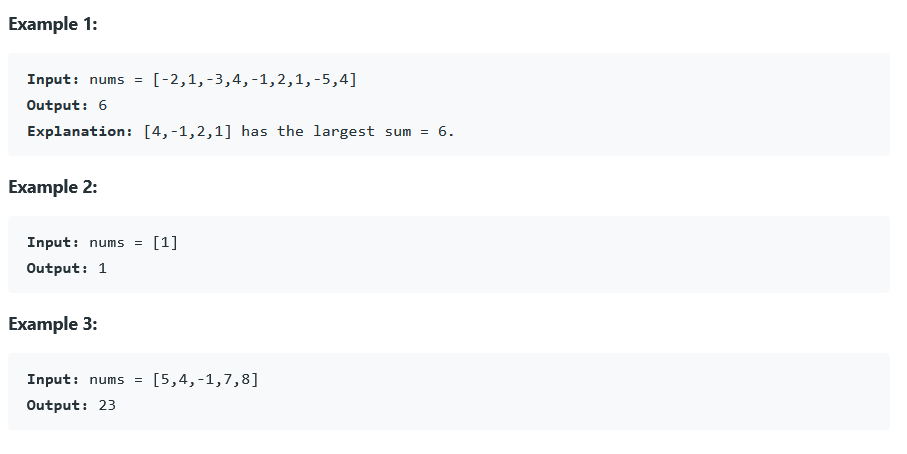
The problem is taken from the below mentioned link:- Leetcode Problem
Approach:-
In most of the case this kind of problems where contiguous subarray is involved can be approached using Kadane’s Algorithm.
Flow-Chart:-
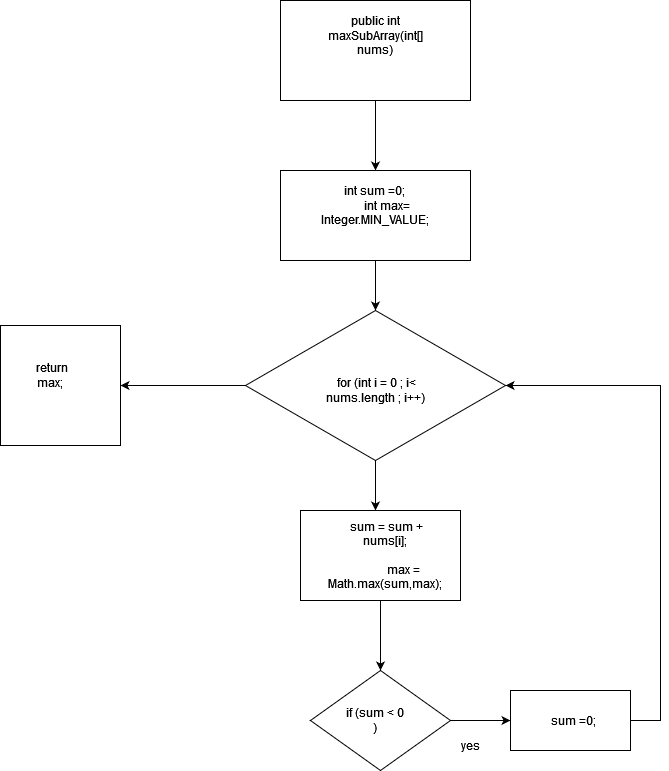
Code Block:-
class Solution {
public int maxSubArray(int[] nums) {
int sum =0;
int max= Integer.MIN_VALUE;
for (int i = 0 ; i< nums.length ; i++) {
sum = sum + nums[i];
max = Math.max(sum,max);
if (sum < 0 ) {
sum =0;
}
}
return max;
}
}
For other problems please visit:- Algorithm Problems and Solutions