Convert Binary Number in a Linked List to Integer
Given head which is a reference node to a singly-linked list. The value of each node in the linked list is either 0 or 1. The linked list holds the binary representation of a number.
Return the decimal value of the number in the linked list.
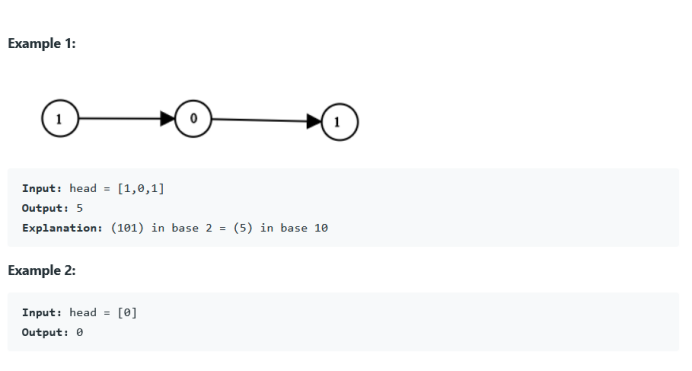
The problem is taken from the below mentioned link:- Leetcode Problem
Approach:-
The simplest approach is using Array List Data Structure. To get the decimal value, the approach is :-
1*(2^2)+0*(2^1)+1*(2^0) = 4 + 0 + 1 = 5
So, store the value in an Array List Data Structure, as :-
1 | 0 | 1 |
Position 0 | Position 1 | Position 2 |
Then using for loop we can achieve our desired result as mentioned below:-
Int p = 0;
for(int i=list.size()-1;i>=0;i–){
int pow = (1<<p); // Finding 2 to the power p
res += (pow*list.get(i));
p++;
}
Flow Diagram:-
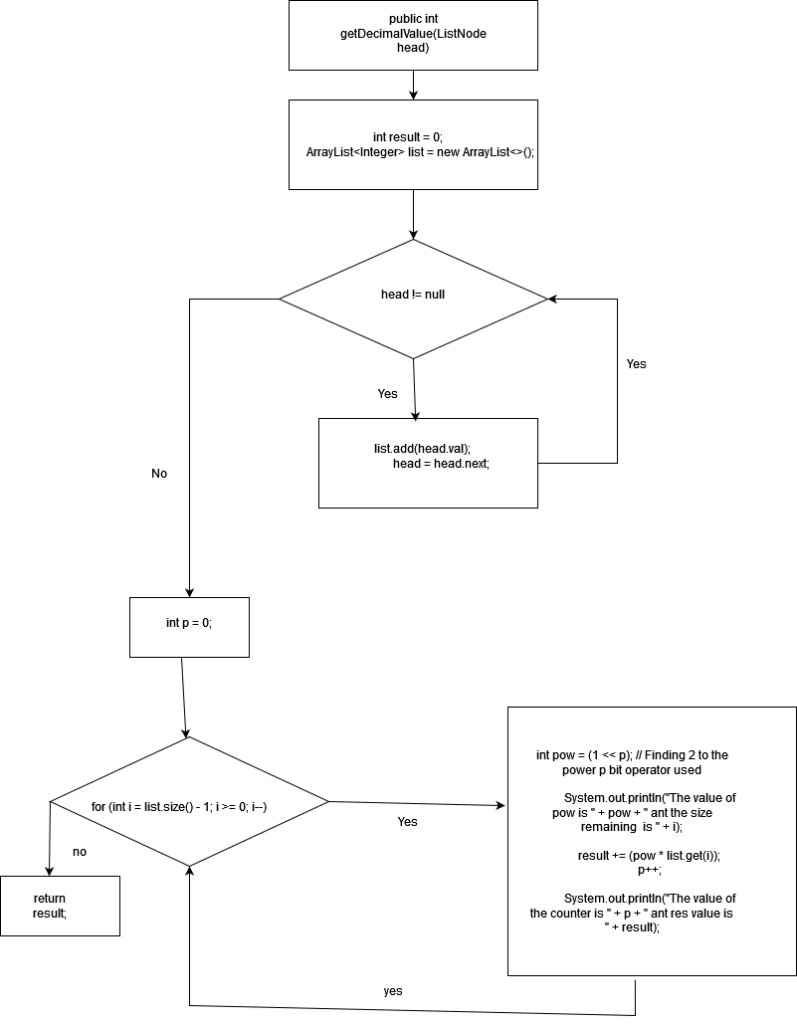
Code:-
public int getDecimalValue(ListNode head) {
int result = 0;
ArrayList<Integer> list = new ArrayList<>();
while (head != null) {
list.add(head.val);
head = head.next;
}
int p = 0;
for (int i = list.size() - 1; i >= 0; i--) {
int pow = (1 << p); // Finding 2 to the power p
System.out.println("The value of pow is " + pow + " ant the size remaining is " + i);
result += (pow * list.get(i));
p++;
System.out.println("The value of the counter is " + p + " ant res value is " + result);
}
return result;
}
For The entire code please follow the below section:-
package ArrayAll;
import java.util.ArrayList;
public class Solution {
public static ListNode head = null;
public static ListNode tail = null;
public static int[] arr;
public static void main(String[] args) {
// TODO Auto-generated method stub
Solution x = new Solution();
x.insertDataAtEnd(1);
x.insertDataAtEnd(0);
x.insertDataAtEnd(1);
x.display();
System.out.println(x.getDecimalValue(head));
}
public int getDecimalValue(ListNode head) {
int result = 0;
ArrayList<Integer> list = new ArrayList<>();
while (head != null) {
list.add(head.val);
head = head.next;
}
int p = 0;
for (int i = list.size() - 1; i >= 0; i--) {
int pow = (1 << p); // Finding 2 to the power p
System.out.println("The value of pow is " + pow + " ant the size remaining is " + i);
result += (pow * list.get(i));
p++;
System.out.println("The value of the counter is " + p + " ant res value is " + result);
}
return result;
}
// method to add new node at the end
public static void insertDataAtEnd(int data) {
/// If only one node present
ListNode newNode = new ListNode(data);
if (head == null) {
head = newNode;
tail = newNode;
}
else {
tail.next = newNode;
tail = newNode;
}
}
public static ListNode display() {
int i = 0;
ListNode current = head;
if (head == null) {
System.out.println("List is empty");
return null;
}
while (current != null) {
System.out.println(current.val + " ");
current = current.next;
}
return current;
}
}
class ListNode {
int val;
ListNode next;
ListNode() {
};
ListNode(int val) {
this.val = val;
}
ListNode(int val, ListNode next) {
this.val = val;
this.next = null;
}
}
For more problem and solutions please visit the below mentioned link:- Algorithms and Data-structure/