Minimum Number of Moves to Seat Everyone
There are n
seats and n
students in a room. You are given an array seats
of length n
, where seats[i]
is the position of the ith
seat. You are also given the array students
of length n
, where students[j]
is the position of the jth
student.
You may perform the following move any number of times:
- Increase or decrease the position of the
ith
student by1
(i.e., moving theith
student from positionx
tox + 1
orx - 1
)
Return the minimum number of moves required to move each student to a seat such that no two students are in the same seat.
Note that there may be multiple seats or students in the same position at the beginning.
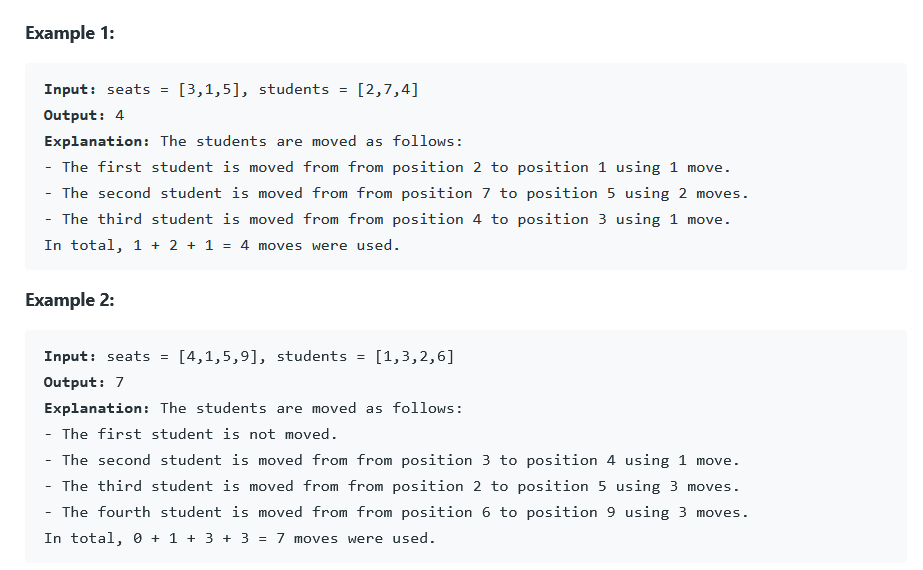
The problem is taken from:- problem statement
Understanding the problem:-
These are the seats number (Available seats current positions) :-
4 | 1 | 5 | 9 |
The students numbers are (Students current positions where they are seating) :-
1 | 3 | 2 | 6 |
Now we have to find the solutions in such a way that, students should be moved from current position to the nearest seat. All the seats must be occupied.
So, the students at position 1 , no need to move.
Students at the position 3, can move to seat 4 or 5 or 9. But the shortest move will be from 3 to 4.
And from 2, it can be moved to position 5. so, need to move (5-2) = 3 position movement
And from 6 it can be moved to 9. 3 position movement.
So, minimum move that can be possible is, = 0 + 1 + 3 + 3 = 7
Approach:-
Best way is sort the two array. And then , for each position find the shortest difference.
So , after sorting the arrays will be:-
These are the seats number (Available seats current positions) :-
1 | 4 | 5 | 9 |
The students numbers are (Students current positions where they are seating) :-
1 | 2 | 3 | 6 |
Now, just find the differences for each positions.
The absolute difference will be the answer.
Code Bock:-
public int minMovesToSeat(int[] seats, int&[] students) {
Arrays.sort(seats);
Arrays.sort(students);
int numberOfMoves = 0;
for (int i = 0; i < seats.length; ++i) {
numberOfMoves += Math.abs(seats[i] - students[i]);
}
return numberOfMoves;
}
For more problems, please visit:-