Count Number of Pairs With Absolute Difference K
Given an integer array nums
and an integer k
, return the number of pairs (i, j)
where i < j
such that |nums[i] - nums[j]| == k
.
The value of |x|
is defined as:
x
ifx >= 0
.-x
ifx < 0
.
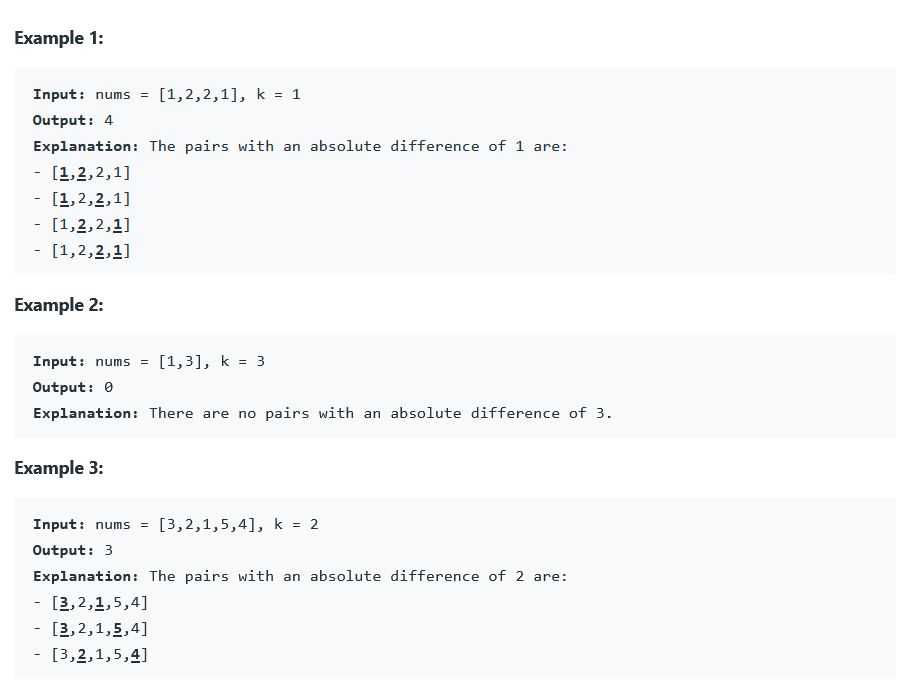
This above problem is taken from :-
count-number-of-pairs-with-absolute-difference-k
Solution :-
Approach:-
Here we will consider one hashMap data structure. Here the values of the array element will be stores as key and the number of occurrence will be stored as value in the given hashMap data structure.
Suppose, the given array is {1,2,2,1} , then finally the map will be as ,
1 2
2 2
As, the value of 1 is present twice in the given array and also the value of 2 is also twice.
Also, two “if ” blocks are used to check the criteria |nums[i] – nums[j]| == k is matching or not.
The flow diagram is mentioned below:-
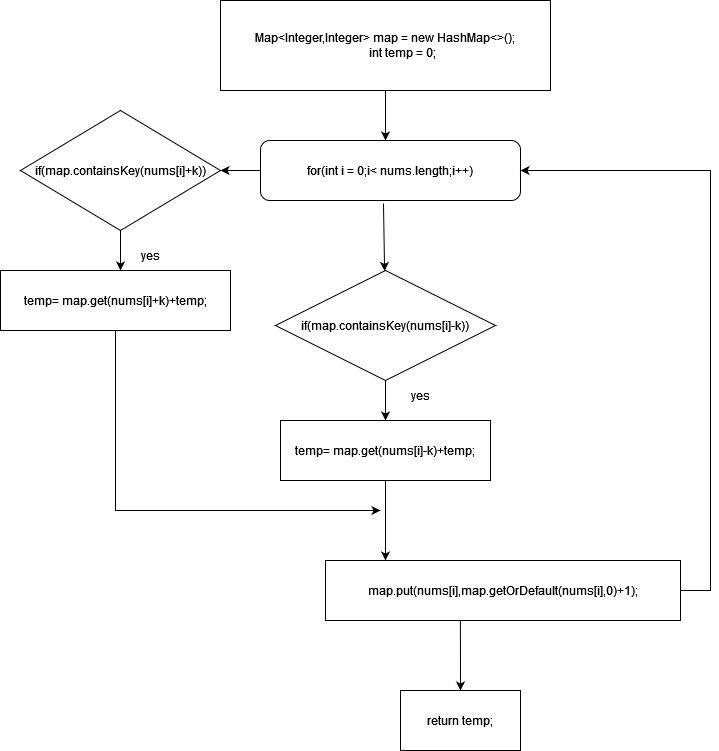
The Code Block:-
class Solution {
public int countKDifference(int[] nums, int k) {
Map map = new HashMap<>();
int temp = 0;
for(int i = 0;i< nums.length;i++){
if(map.containsKey(nums[i]-k)){
temp= map.get(nums[i]-k)+temp;
}
if(map.containsKey(nums[i]+k)){
temp= map.get(nums[i]+k)+temp;
}
map.put(nums[i],map.getOrDefault(nums[i],0)+1);
}
return temp;
}
}
For other solutions, please visit:-