Substrings of Size Three with Distinct Characters
A string is good if there are no repeated characters.
Given a string s
, return the number of good substrings of length three in s
.
Note that if there are multiple occurrences of the same substring, every occurrence should be counted.
A substring is a contiguous sequence of characters in a string.
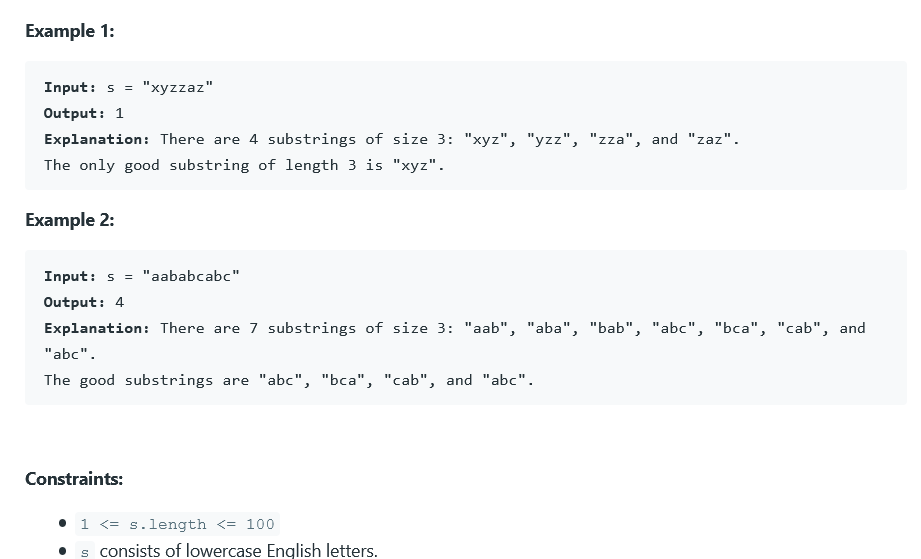
For complete problem description please visit substrings-of-size-three-with-distinct-characters
Solution:-
Flow Diagram:-
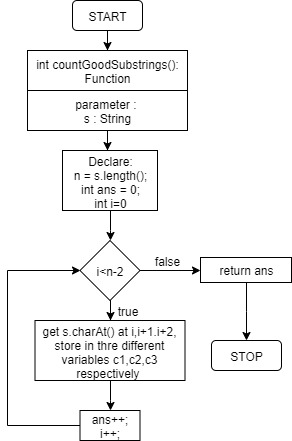
The logic is, as it was already mentioned that length will be three. So we are comparing only the three characters.
Code Block:-
class Solution { public int countGoodSubstrings(String s) { int n = s.length(); int ans = 0; // Here loop will go upto (n-2) as we are comparing (i+1) and //(i+2) position with (i) for(int i = 0; i < n - 2; i++) { char c1 = s.charAt(i); char c2 = s.charAt(i + 1); char c3 = s.charAt(i + 2); if(c1 == c2 || c2 == c3 || c1 == c3) continue; ans++; } return ans; } }
For others problems please visit algorithms-and-datastructure