How many numbers are smaller than the current number:-
Given the array nums
, for each nums[i]
find out how many numbers in the array are smaller than it. That is, for each nums[i]
you have to count the number of valid j's
such that j != i
and nums[j] < nums[i]
.
Return the answer in an array.
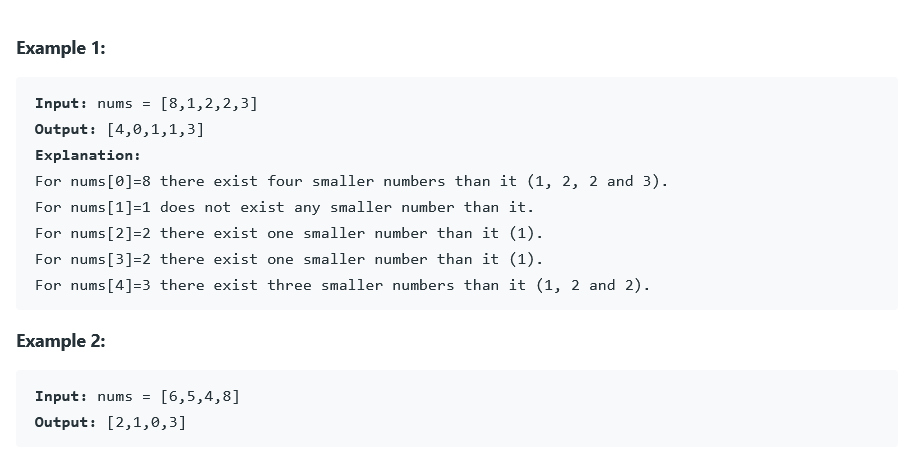
To find the original problem, please visit LeetCode Problem.
Solution:-
Flow Diagram:-
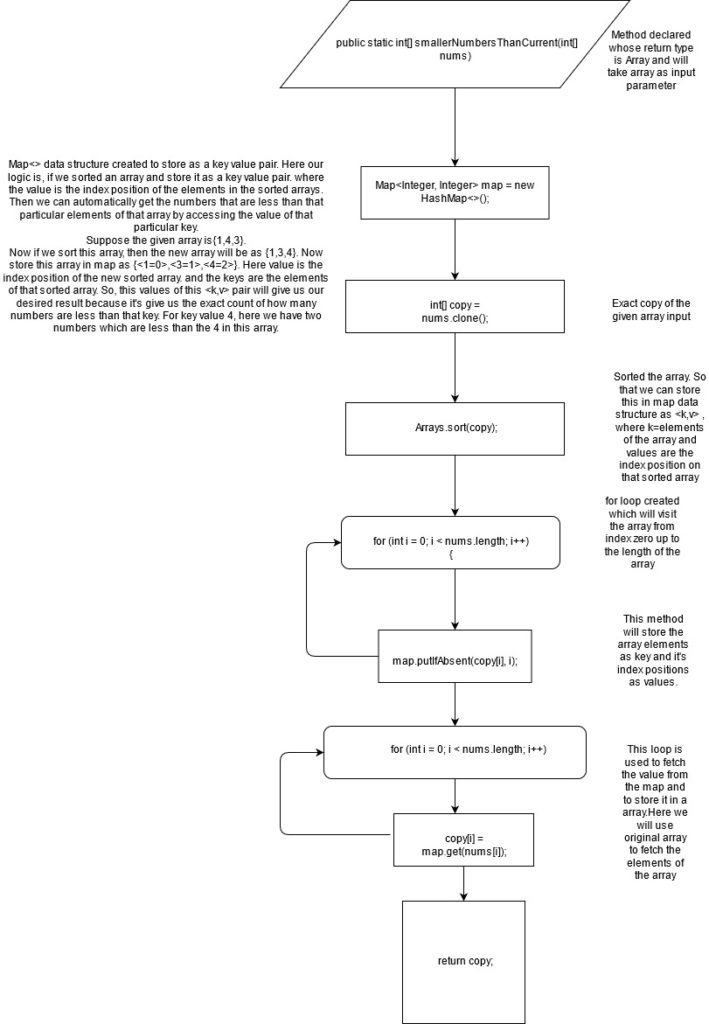
Sample Code in Java:-
package test;
import java.util.Arrays;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
public class SolutionNew {
public static int[] smallerNumbersThanCurrent(int[] nums) {
//Map Database
Map map = new HashMap<>();
int[] copy = nums.clone();
Arrays.sort(copy);
for (int i = 0; i < nums.length; i++) {
map.putIfAbsent(copy[i], i);
}
for (int i = 0; i < nums.length; i++) {
System.out.println("The nums value is " + Arrays.toString(nums));
copy[i] = map.get(nums[i]);
}
return copy;
}
public static void main(String[] args) {
int[] numbers = { 1, 9, 3 };
}
}
For other LeetCode Problems and Solution please visit:- algorithms-and-datastructure Section.