What is Page Object Model?
It’s a design pattern , which is used by many test automation projects. The Page Object is basically a class file which acts as an interface of any web page. This class contains the web elements and many methods to interacts with those web-elements. And to interact with these pages, we just create the object of this page object class from different test class and then calls the methods of this page object class.
Example:-
Suppose we want to automate the login functionality of rediffMail Website. So for that we have to perform the following actions on that Rediff login Page.
- Action 1: Enter the Username.
- Action 2: Enter the Password.
- Action 3: Click on the Sign in Button.
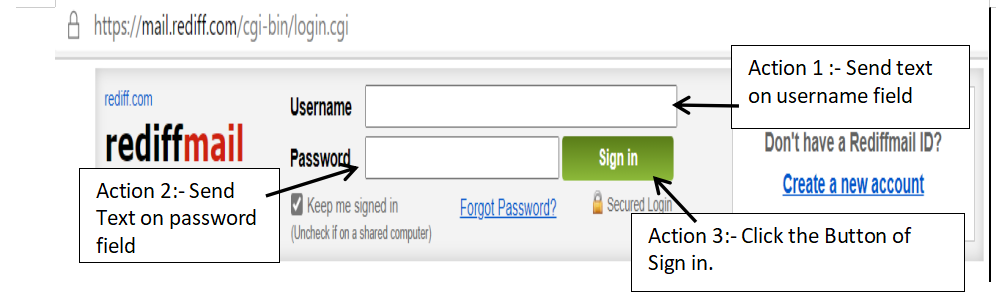
Now if we follow the Page Object Design Pattern, then we will create one class of this login page, which contains all the methods whose return types are WebElement of this page. And these methods can be used by other class as we have made those methods as public. One Sample Page Object Class for this Login page is mentioned below:-
public class RediffLoginPage {
public WebDriver driver;
//Constructor to pass the driver from test class
public RediffLoginPage(WebDriver driver) {
this.driver = driver;
}
//user name selector
By userName = By.name("login");
//Password selector
By passwd = By.id("password");
//Sign in button Seletor
By signIn = By.xpath("[value='Sign in']");
//return webelement method for username
//This method is public so that this webelement of this Login page can
//be used by different test class
public WebElement userName() {
return driver.findElement(userName);
}
//return webelement method for password
//This method is public so that this webelement of this Login page can
//be used by different test class
public WebElement passWord() {
return driver.findElement(passwd);
}
//return webelement method for sign in Button
//This method is public so that this webelement of this Login page can
//be used by different test class
public WebElement signIN() {
return driver.findElement(signIn);
}
}
So , what we did basically, created one class that acting as an interface via which the webelements of that page can be accessed. Please check the pictorial presentation for better understanding:-
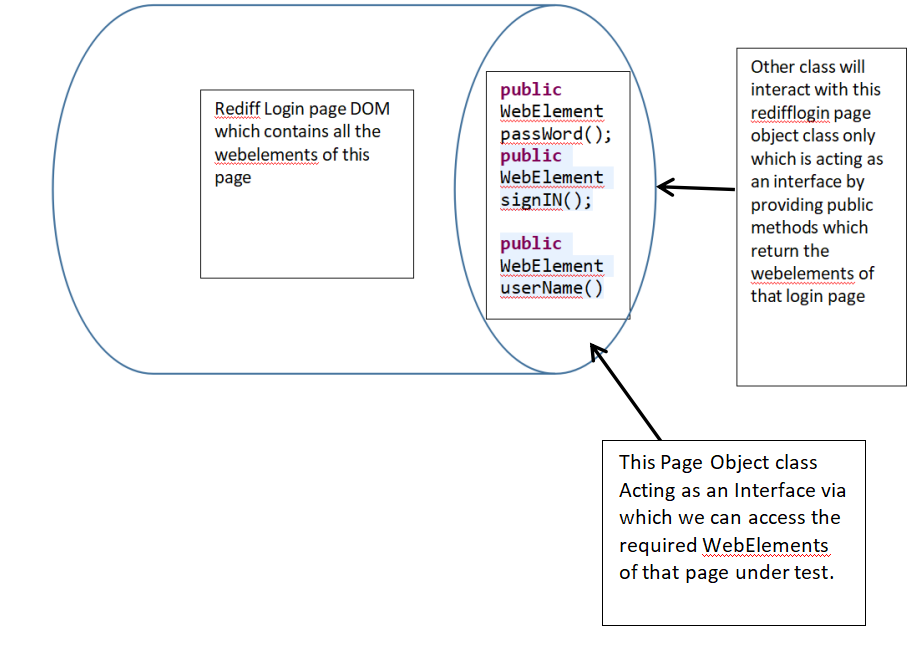
Now To perform the test on that page, please check the following code , where we just called the methods to perform a specific actions on those webElements. Sample Codes are mentioned below:-
public class RediffLoginPageTest {
@Test
public void RediffLoginTestcase() {
System.setProperty("webdriver.chrome.driver" , "H:\\chromedriver_win32\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
driver.get("https://mail.rediff.com/cgi-bin/login.cgi");
RediffLoginPage loginPageObject = new RediffLoginPage(driver);
//Action 1:- Enter the Username
//We are only calling the methods from pageobject class which is returning the webelemnts
// We are not defining any webelemnts inside this test class
loginPageObject.userName().sendKeys("username");
//Action 2:- Enter the Password.
loginPageObject.passWord().sendKeys("password");
//Action 3:- Click on the Sign in Button.
loginPageObject.signIN().click();
}
}
So Just Looking at the test class we can tell the advantages of this Page Object Design Pattern. The Advantages of this Design are mentioned below:-
Advantages of Page Object Model:-
- Reusability:- We can reuse this page object class from any test classes. We don’t need to mention the webelements of this particular page in different test classes. Just make an object of this class and call the methods that returns the web elements, That’s it as we have done here.
- Maintainability:- As we can see from the above sample code, if webelements are changed by the developers, then only we have to amend the pageobject class. So it’s very easy to maintain the code properly.
- Readability:- And readability of the Test class improves a lot as we are reusing the webelements which are defined inside the page object class.
Project Hierarchy:-
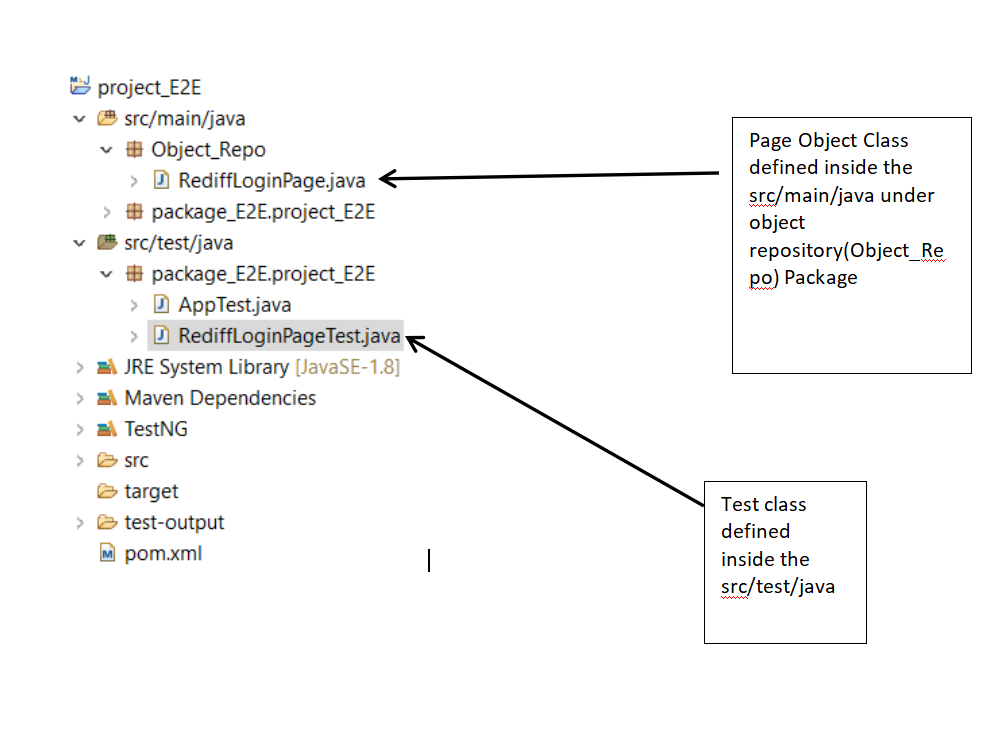
Nice One