As we are aware that the ArrayLists in Java stores the pointers to objects. Then how can I store the primitive datatypes inside the ArrayLists. Because when storing the int type in ArrayLists it’s showing the below mentioned error in IDE.

Same thing will happen for data type double also as shown below:-

So, here one thing we can do that we can define one class in a such a way that it will behave like int datatype. int datatype actually stores a value and returns that value when called upon. Similarly we can define a class as mentioned below, which will store a int value and return to us when the appropriate method will be called.
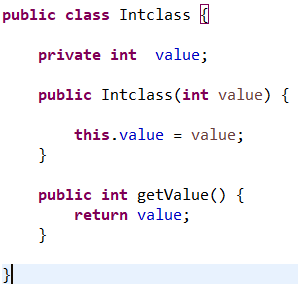
So, here we are wrapping int value in a class. Please see my code of Arraylist_ex1 class. Where I am storing the int value inside the Arraylists by instantiating the class with passing one new value. That’s actually the AUTOBOXING. When we are creating one object of the Intclass with a new int value, then in Java actually we are creating a new objects of Intclass with new int value. So we boxed int datatype inside a class.
And we can call that element from the lists by calling the getter method of the Intclass, as shown below, which is nothing but UNBOXING.
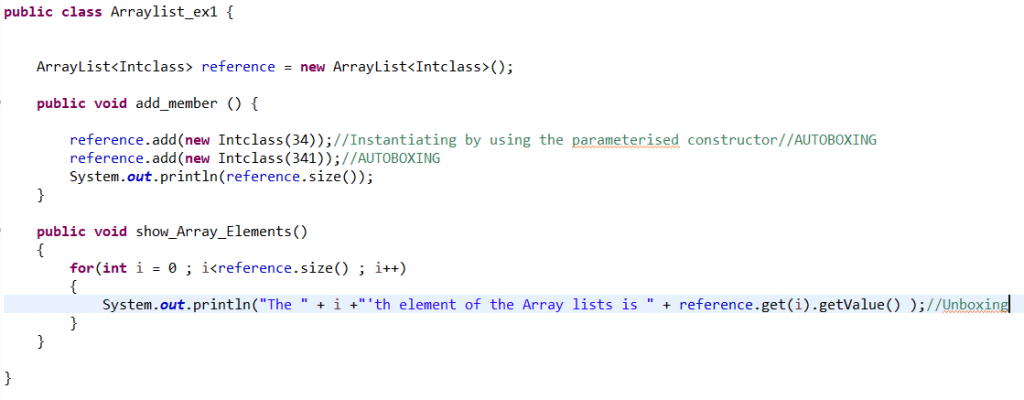
Java provides one built in class for storing primitive datatypes within the ArrayLists. For int datatype it’s “Integer”. This is a wrapper class only. So using the Integer class the code will look like as mentioned below:-
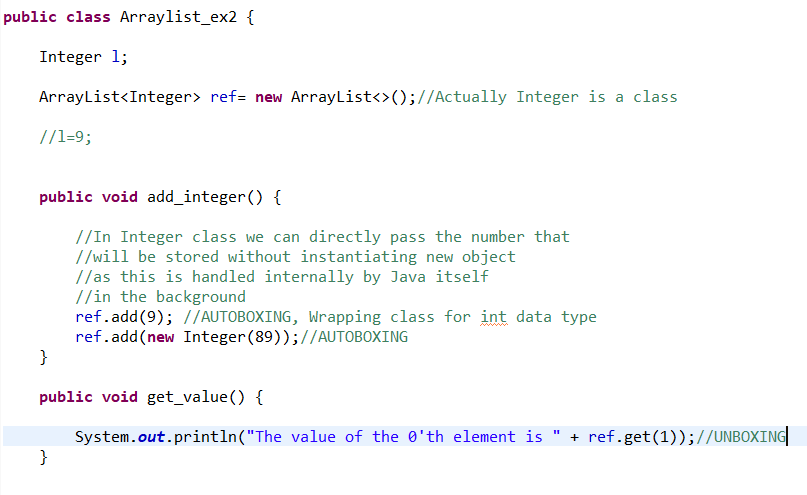
It’s always better to use these wrapper class for primitive data tyeps instead of defining your own wrapper class .