Here we will randomly select the name from list of names, and randomly prizes will be assigned to them. So lets write the outline of the code.
Load list of Names
Load the prizes list
Then randomly select the names and assign them to a variable
Then randomly select the prizes and assign them to a variable
Now print the names with the prize they have won.
Then ask the user whether they want to quit or play
If they want to play then continue else quits.
Now let’s check the code:-
import sys,random
print("Welcome to Lottery competetion")
while True:
Name = ('John Lee','Tom Bilbow','Jacky Lee','Hogun Tom','Chow lee','Cherry Sawn')
Prize = ('Car','Bike','1 Billion Dollar','Pen')
Winner = random.choice(Name)
Won = random.choice(Prize)
print(f'{Winner} won the {Won} in the Lottery',file=sys.stderr)
print("\n\n")
user_input = input ("Want to play again then press enter else 'n' to quit\n")
if user_input.lower() =='n':
break
input("Press enter to exit")
This code will work, now we have to check whether the code design is correct or not, which can be checked using Pylint. In windows install the pylint using pip install and then run it as follows to check the error in the code:-
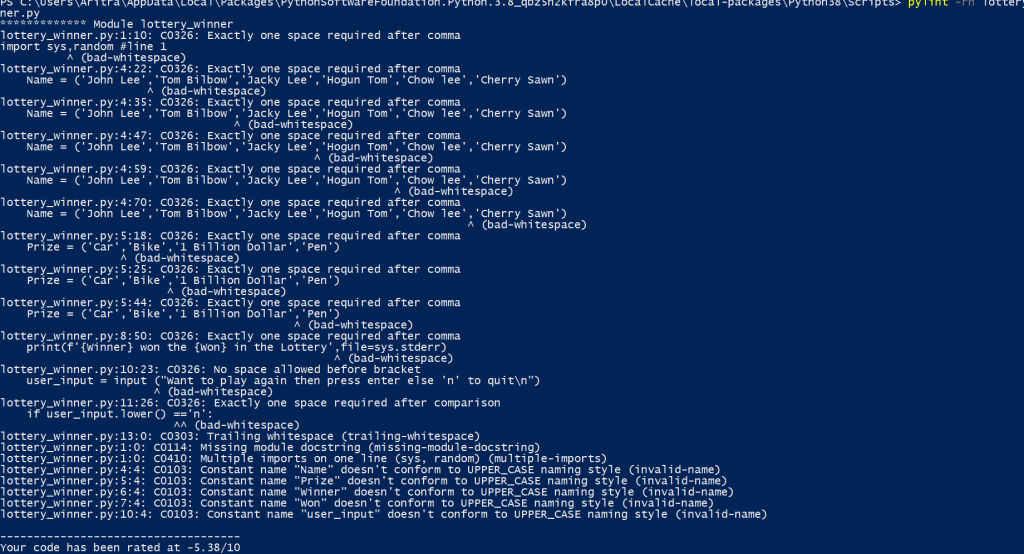
So as mentioned above, lots of error are present in the code. So we have to correct those. Now the corrected code is mentioned below. Always make it a practice to write your code according to the design that is followed by the international community so that your code will be much more readable.
"""Lottery Winner Game"""
import sys
import random #line 1
def main():
"""This is the main Function"""
print("Welcome to Lottery competetion")
while True:
winner_name = ('John Lee', 'Tom Bilbow', 'Jacky Lee', 'Hogun Tom',
'Chow lee', 'Cherry Sawn')
prize_name = ('Car', 'Bike', '1 Billion Dollar', 'Pen')
winner_name = random.choice(winner_name)
prize_name = random.choice(prize_name)
print(f'{winner_name} won the {prize_name} in the Lottery', file=sys.stderr)
print("\n\n")
user_input = input("Want to play again then press enter else 'n' to quit\n")
if user_input.lower() == 'n':
break
input("Press enter to exit")
if __name__ == "__main__":
main()
