Iterator is a method which returns an iterator over elements. It is defined inside the “Iterable” interface of java collection framework. The source code of this interface can be found in the below mentioned link.
Let’s create on collection of hotels as mentioned in the below mentioned code:-
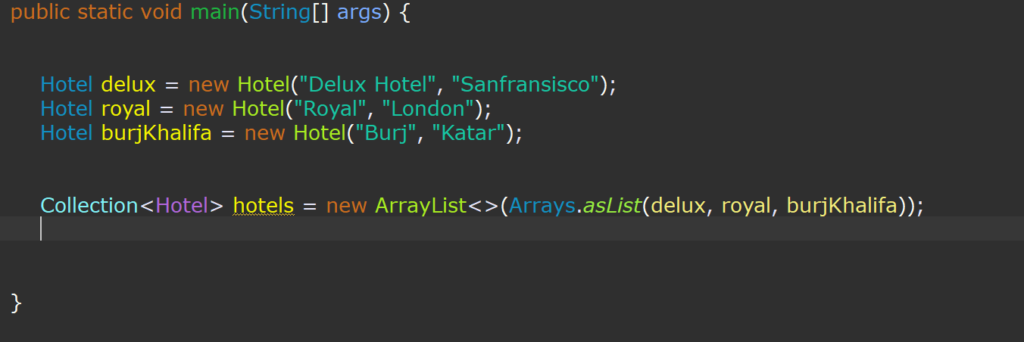
The source code of the “Hotel” class is mentioned below:-
public class Hotel {
private String name;
private String location;
//constructor with fields
public Hotel(String name, String location) {
this.name = name;
this.location = location;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getLocation() {
return location;
}
public void setLocation(String location) {
this.location = location;
}
}
As already mentioned that iterator method returns iterator, so create an iterator variable as mentioed below:-

Now to fetch the next piece of elements from the collection we can use the “next()” method. next method is part of the Iterator interface and returns the next element in the iteration. Each time we are using the “next()” method, the loop is going to advance one position forward. As here we have used the generic type “Hotel”, so the “next()” method will return the object of type “Hotel”.
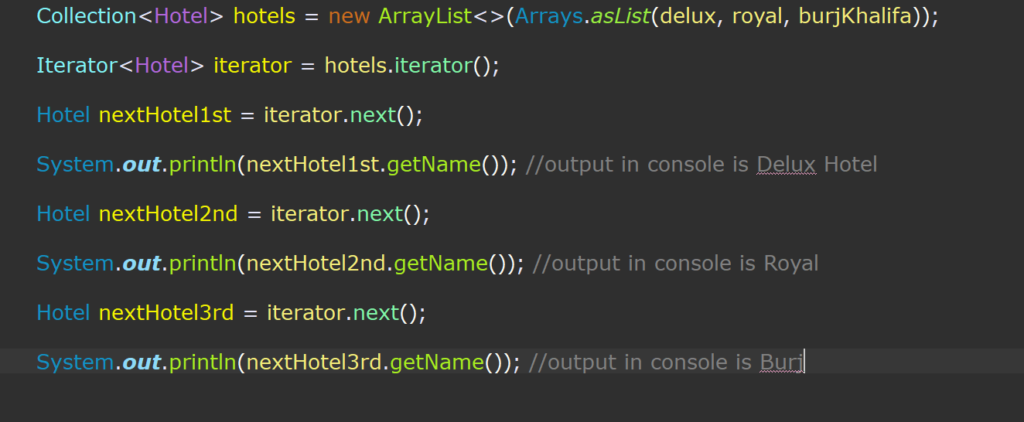
But in real time we generally use while loop to iterate over the collection as mentioned below. This was the popular approach to traverse through the collection when the for-each loop was not introduced. The code block is mentioned below:-
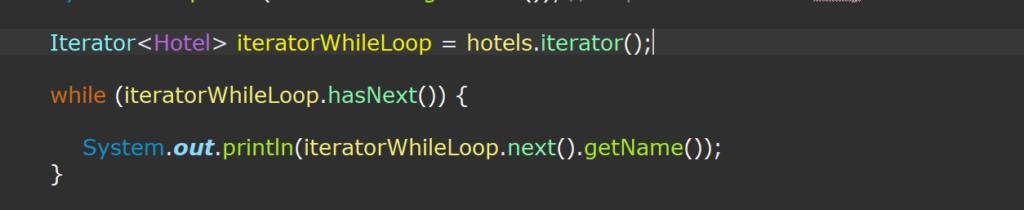
We can also use the for-each loop to traverse over the collection as mentioned below :-
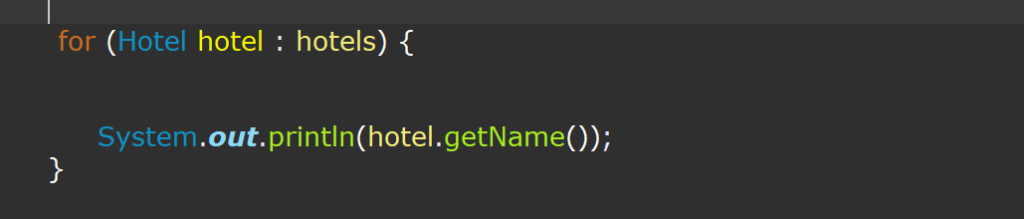
The complete code of the main method class is mentioned below:-
public static void main(String[] args) {
Hotel delux = new Hotel("Delux Hotel", "Sanfransisco");
Hotel royal = new Hotel("Royal", "London");
Hotel burjKhalifa = new Hotel("Burj", "Katar");
Collection<Hotel> hotels = new ArrayList<>(Arrays.asList(delux, royal, burjKhalifa));
Iterator<Hotel> iterator = hotels.iterator();
Hotel nextHotel1st = iterator.next();
System.out.println(nextHotel1st.getName()); //output in console is Delux Hotel
Hotel nextHotel2nd = iterator.next();
System.out.println(nextHotel2nd.getName()); //output in console is Royal
Hotel nextHotel3rd = iterator.next();
System.out.println(nextHotel3rd.getName()); //output in console is Burj
Iterator<Hotel> iteratorWhileLoop = hotels.iterator();
while (iteratorWhileLoop.hasNext()) {
System.out.println(iteratorWhileLoop.next().getName());
}
for (Hotel hotel : hotels) {
System.out.println(hotel.getName());
}
2 Replies to “How to access collection elements in java using iterator method?”
Comments are closed.