Contains Duplicate
Given an integer array nums
, return true
if any value appears at least twice in the array, and return false
if every element is distinct.
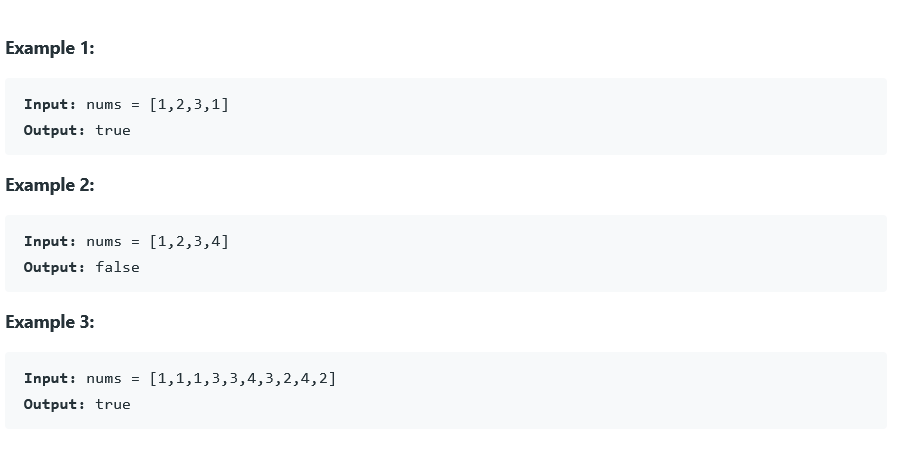
The above problem is taken from the below mentioned link:- Leetcode Problem
Approach:-
Use of HashSet data structure will solve the problem. Here Set is chosen as it will not store duplicate data. Now, if the length of the given array is same with the size of the SET data structure then here all the values in the given array is unique, other wise the value will always be different if array contains the duplicate elements.
Flow-Diagram:-
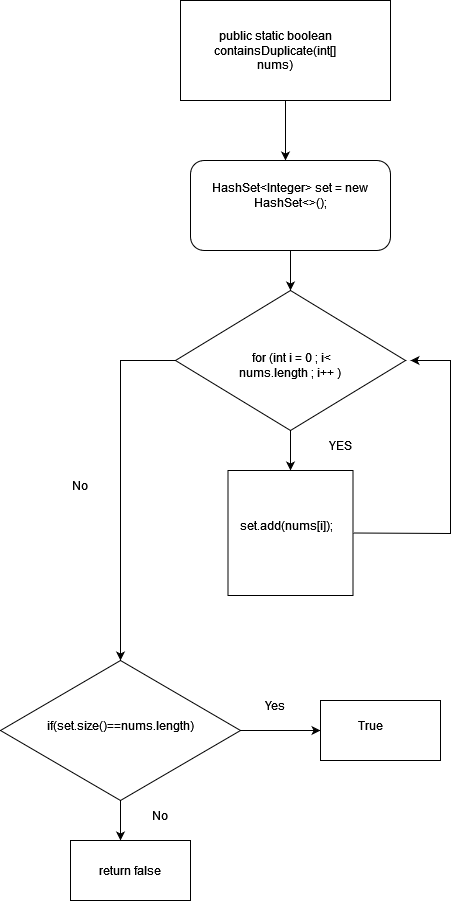
Code block:-
class Solution {
public boolean containsDuplicate(int[] nums) {
HashSet set = new HashSet<>();
for (int i = 0 ; i< nums.length ; i++ ) {
set.add(nums [i]);
}
if(set.size()==nums.length) {
return false;
}
else { return true;}
}}
For more problems and solutions please visit:- Algorithm problems