This is a very common interview questions asked by many interviewer in SDET and SET interviews. At the very first, clear with the interviewer about the characters that the given string can hold.
Here we are assuming that the string can contain ASCII characters with value from 1 to 128.
Aproach:-
So, at the very first line, we will check the length of the string. If, it’s greater than 128, then the program will return false.
Then we will create one array which can contain only the boolean values as follows:-

Here, the default value is false. So, this array will look like as,
Value of the array | false | false | false | false | false |
Index position | 65 | 66 | 67 | 68 | 69 |
Now, one for loop is created which will iterate over the given string and will check each characters of the given String. So, the number of iteration must be less than the length of the given string.

Now each characters are converted to its numeric ASCII values and is stored in a variable.

Suppose, our given String is = “AA”
So, when i = 0, the Character is “A”. The ASCII value of uppercase “A” is 65. So for i=0, we will set the value true at index position 65 for the given array. The code block is mentioned below:-
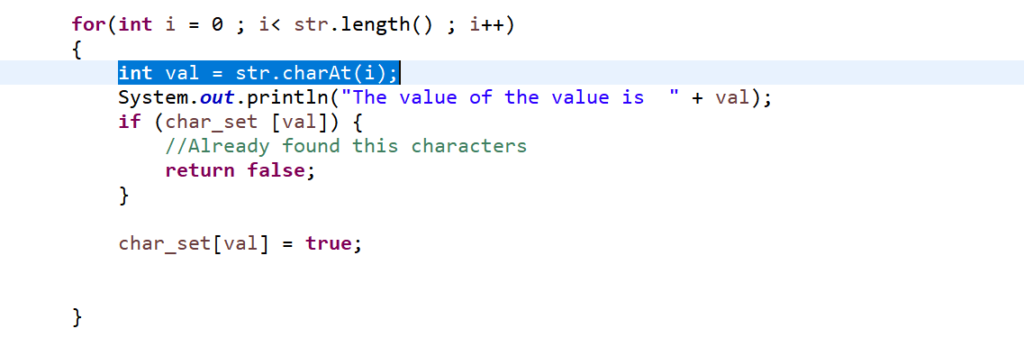
Now, after first iteration the array will look like,
Value of the array | true | false | false | false | false |
Index position | 65 | 66 | 67 | 68 | 69 |
Now, in the second iteration, when i = 1, it will found that at the index postion 65, the value of the array block is already true, so it will return false.
Code Block :-
public class Example1 {
//One solution is create a array of boolean characters.
// Index position is determined by i. Now if , the same character comes at
// any other position then simply we will return the false value
public static boolean ifUniqueCharacter(String str) {
//Considering the ASCII String
if (str.length() > 128)
return false;
//One boolean array is taken
boolean [] char_set = new [128];
System.out.println("The default value is " + char_set[0]);
for(int i = 0 ; i< str.length() ; i++)
{
int val = str.charAt(i);
System.out.println("The value of the value is " + val);
if (char_set [val]) {
//Already found this characters
return false;
}
char_set [val] = true;
}
return true;
}
public static void main(String[] args) {
// TODO Auto-generated method stub
System.out.println(ifUniqueCharacter("AA"));
}
//The time complexity is O(n) and space complexity is O(1).
//as here the size of iteration will depend on the size of the array
//and only one data structure is used which is array
}
Please correct the "[]" and "<" and ">" sign before copying the code.