Lambda expression is used for the implementation of the functional interfaces. Functional interface is those interfaces which has only one abstract method. We can declare an interface as functional interface by using the annotation @FunctionalInterface. e.g. Comparator interface in java is a functional interface. Please visit the official site for more information:-
Example 1:-
One interface is created with one single abstract method.
Without lambda expression:-
interface Iexampleinterface { // One method with no arguments public void printName(); } public class LambdaExamples { public static void main(String[] args) { // Anonymous class // When we are initializing anonymous class, then automatically the method will // be implemented Iexampleinterface example = new Iexampleinterface() { @Override public void printName() { System.out.println("Printing My Name"); } }; example.printName(); } }
With Lambda Expression:-
@FunctionalInterface
interface Iexampleinterface {
// One method with no arguments
public void printName();
}
public class LambdaExamples {
public static void main(String[] args) {
// Anonymous class
// When we are initializing anonymous class, then automatically the method will
// be implemented
/*
* @Override public void printName() {
*
* System.out.println("Printing My Name"); } };
*/
// () is the argument part of Lambda function
// -> Arrow sign
//And in the body part, print statement has been added
Iexampleinterface example = () -> System.out.println("Printing My Name");
example.printName();
}
}
Code has become very clear and concise after using the lambda expression.
Example 2:-
Java Lambda expression example when single parameter is used.
@FunctionalInterface interface Iexampleinterface { // One method with single arguments public void printName(String name); } public class LambdaExamples { public static void main(String[] args) { // Anonymous class // When we are initializing anonymous class, then automatically the method will // be implemented /* * @Override public void printName() { * * System.out.println("Printing My Name"); } }; */ // () is the argument part of Lambda function // -> Arrow sign //And in the body part, print statement has been added Iexampleinterface example1 = (name) -> System.out.println("Printing My Name :" + name); //Another way of calling it with out using parenthesis Iexampleinterface example2 = name -> System.out.println("Printing My Name and using lambda expression in a different way :" + name); //Multi-line body of Lambda expression Iexampleinterface example3 = name -> {System.out.println("Printing My Name and using lambda expression with multiline body :" + name); System.out.println("This is line 2"); } ; example1.printName("Evan1"); example2.printName("Evan2"); example3.printName("Evan3"); } }
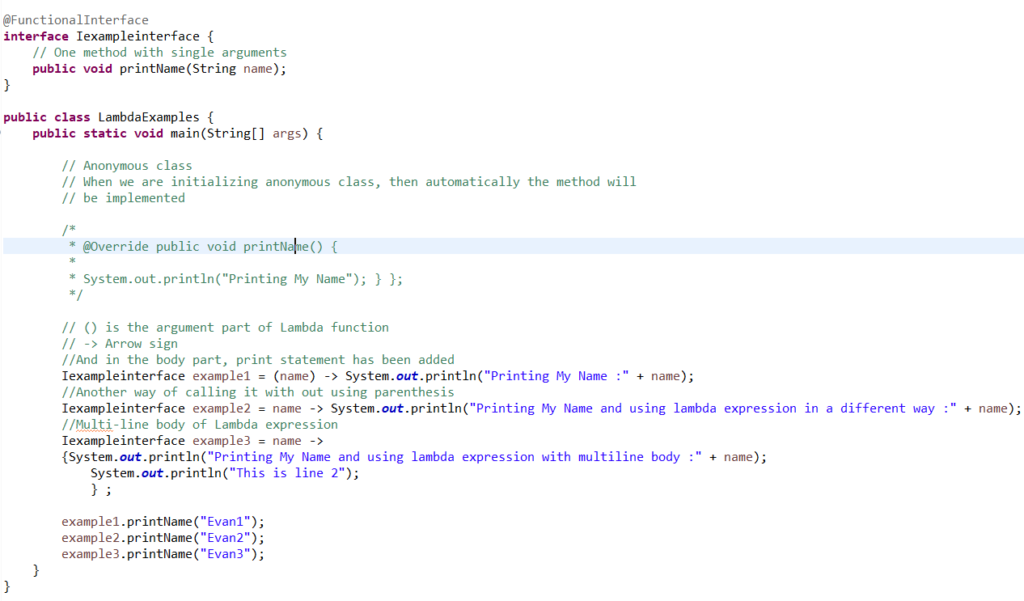
Example 3:-
When the abstract method within the functional interface has multiple arguments:-
@FunctionalInterface interface Iexampleinterface { // One method with multiple arguments public void printName(String firstName, String lastName); } public class LambdaExamples { public static void main(String[] args) { // Anonymous class // When we are initializing anonymous class, then automatically the method will // be implemented /* * @Override public void printName() { * * System.out.println("Printing My Name"); } }; */ // () is the argument part of Lambda function // -> Arrow sign //And in the body part, print statement has been added Iexampleinterface example1 = (firstName, surName) -> System.out.println("Printing My Full Name : " + firstName + " " + surName); //Multi-line body of Lambda expression Iexampleinterface example2 = (firstName, surName) -> {System.out.println("Printing My First Name and using lambda expression with multiline body : " + firstName); System.out.println("Printing My surname and using lambda expression with multiline body : " + surName); } ; example1.printName("Evan", "Bell" ); example2.printName("Jack" , "johnas"); } }
The picture of this code block:-
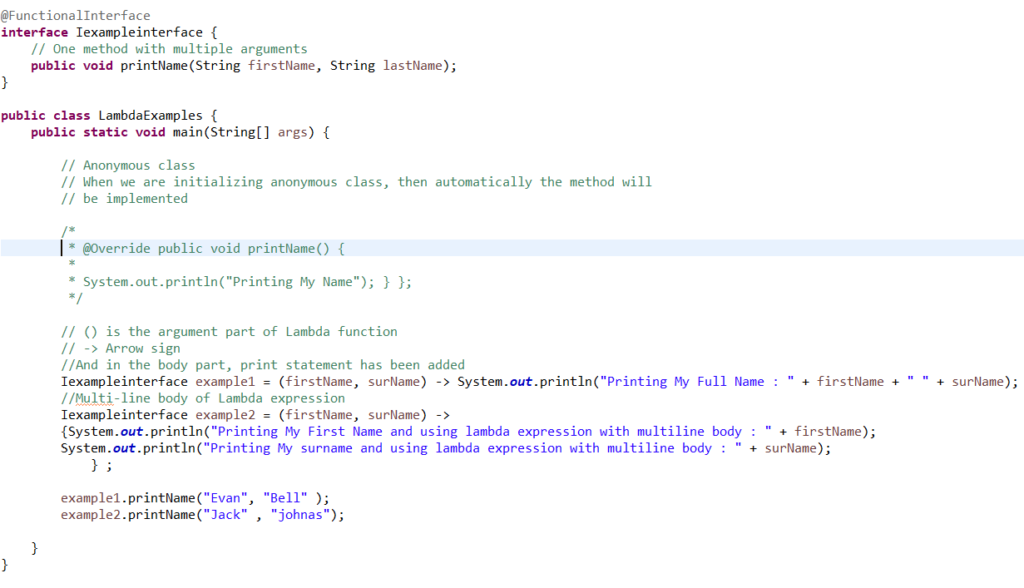
The lambda expression is used to sort an Array as mentioned in the below example:-