Check if All the Integers in a Range Are Covered
You are given a 2D integer array ranges
and two integers left
and right
. Each ranges[i] = [starti, endi]
represents an inclusive interval between starti
and endi
.
Return true
if each integer in the inclusive range [left, right]
is covered by at least one interval in ranges
. Return false
otherwise.
An integer x
is covered by an interval ranges[i] = [starti, endi]
if starti <= x <= endi
.
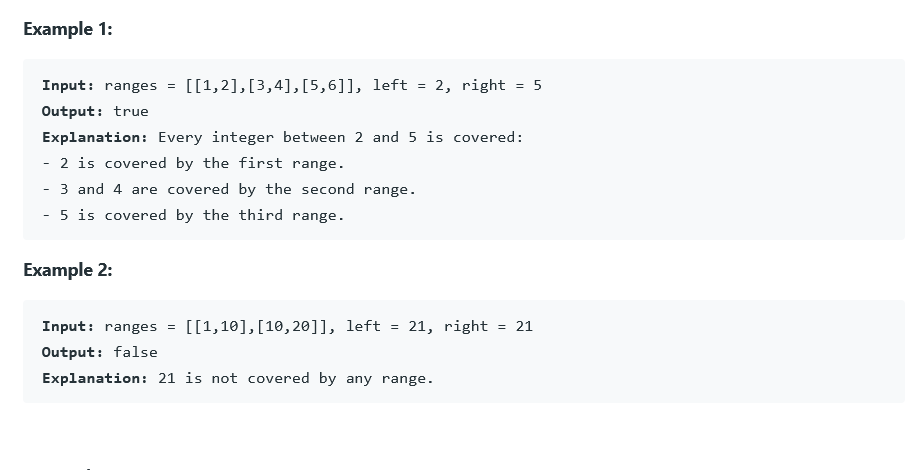
Solution :-
Flow Diagram:-
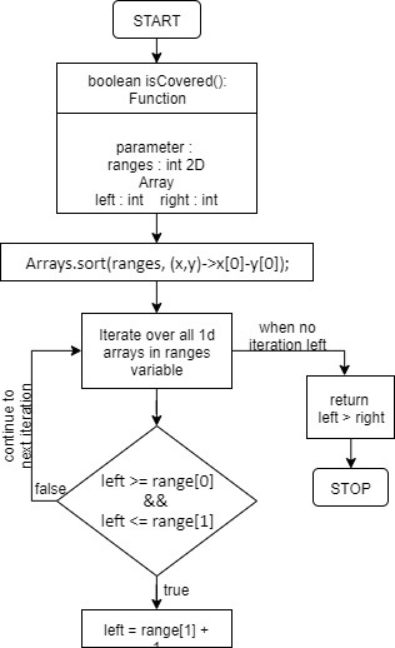
Code Block:-
public boolean isCovered(int[][] ranges, int left, int right) { //lambda function used to sort the array Arrays.sort(ranges, (int[] x, int[] y) -> x[0]-y[0] ); //Printing the sorted array for (int i = 0; i < ranges.length; i++) { System.out.println(Arrays.toString(ranges[i])); } for(int[] range: ranges) { if(left >= range[0] && left <= range[1]) left = range[1] + 1; } return left > right; }
To know more about array sorting using Lambda Function, please visit the site:-
java-sort-array-of-objects-in-ascending-and-descending-order-using-java8-lambda
For more leetcode problem and solutions please visit algorithms-and-datastructure