Check if Word Equals Summation of Two Words
The letter value of a letter is its position in the alphabet starting from 0 (i.e. 'a' -> 0
, 'b' -> 1
, 'c' -> 2
, etc.).
The numerical value of some string of lowercase English letters s
is the concatenation of the letter values of each letter in s
, which is then converted into an integer.
- For example, if
s = "acb"
, we concatenate each letter’s letter value, resulting in"021"
. After converting it, we get21
.
You are given three strings firstWord
, secondWord
, and targetWord
, each consisting of lowercase English letters 'a'
through 'j'
inclusive.
Return true
if the summation of the numerical values of firstWord
and secondWord
equals the numerical value of targetWord
, or false
otherwise.
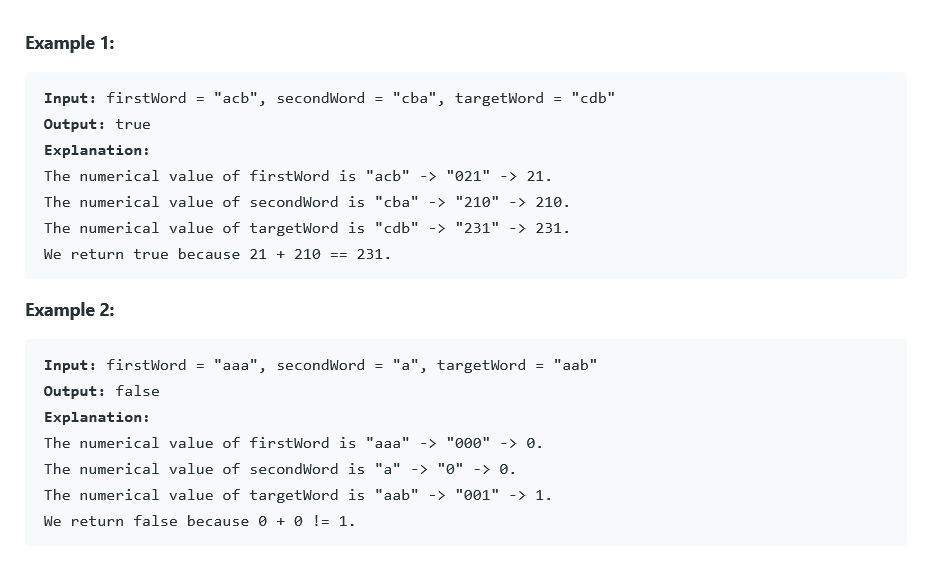
For detail problem description please visit check-if-word-equals-summation-of-two-words
Solution:-
Flow Diagram:-
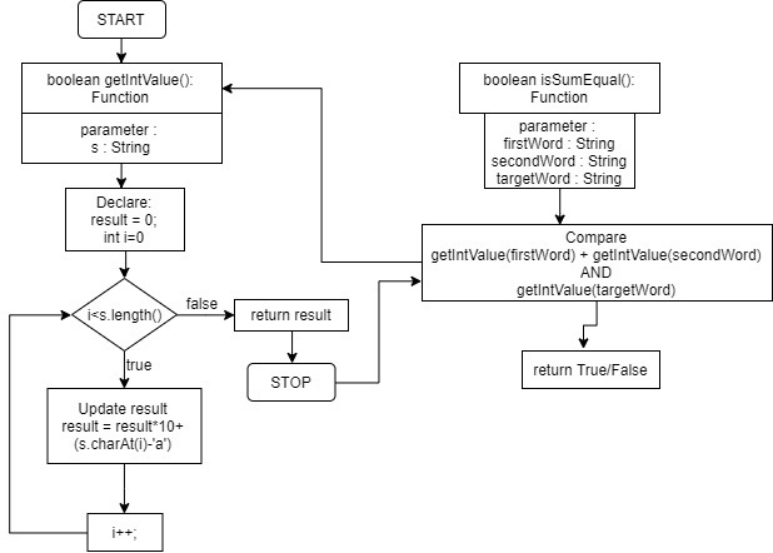
Code Block:-
public class SolutionTest { public static boolean isSumEqual(String firstWord, String secondWord, String targetWord) { return getIntValue(firstWord) + getIntValue(secondWord) == getIntValue(targetWord); } private static int getIntValue(String s) { int result = 0; for(int i=0;i<s.length();i++) { result = result * 10 + (s.charAt(i) - 'a'); System.out.println((s.charAt(i) - 'a'));} return result; } public static void main(String[] args) { String firstWord = "aaav"; String secondWord = "aa"; String thirdWord = "a"; System.out.println( isSumEqual(firstWord,secondWord,thirdWord)); //The result should be false } }
For other problems please visit algorithms-and-datastructure.